- Published on
Java Class Loader
- Authors
- Name
Introduction
Java Class Loaders are responsible for locating, loading and initializing classes at runtime. This gives Java an unmatched level of flexibility and extensibility. When running an application, the JVM doesn't eagerly load all the classes at once. Instead, it loads classes on demand, as they are referenced by other classes being executed. This is an optimization technique that reduces memory footprint and startup time.
Class Loader Hierarchy
The Class Loader is organized in a hierarchy. It is a delegation model, where each class loader has a parent class loader. There are three types of class loaders:
- Bootstrap class loader
- Extensions class loader
- System class loader
Bootstrap class loader
The Bootstrap class loader is the root of the class loader hierarchy. Its responsibility is to load the core Java classes, JVM internal classes and native libraries. This is not a Java class, but a native implementation. Classes are loaded from the jre/lib
directory.
Extensions class loader
The Extensions class loader sits in the middle of the hierarchy. It is responsible for loading classes from the Java extensions directory, jre/lib/ext
. It handles optional libraries and extensions that enhance the core Java platform.
System class loader
The System class loader is the leaf of this tree structure. System class loader loads classes from the classpath. It is also known as the Application class loader. The classpath is a list of JAR files and directories that the JVM scans to find classes. The classpath can be set using the -classpath
or -cp
option when starting the JVM or by setting the CLASSPATH
environment variable.
Class Loader Delegation Model
The Class Loader hierarchical model guarantees that classes are loaded only once. A class loader first delegates the class loading to its parent class loader before attempting to load the class itself. The parent class loader will delegate the class loading to its parent class loader and so on.
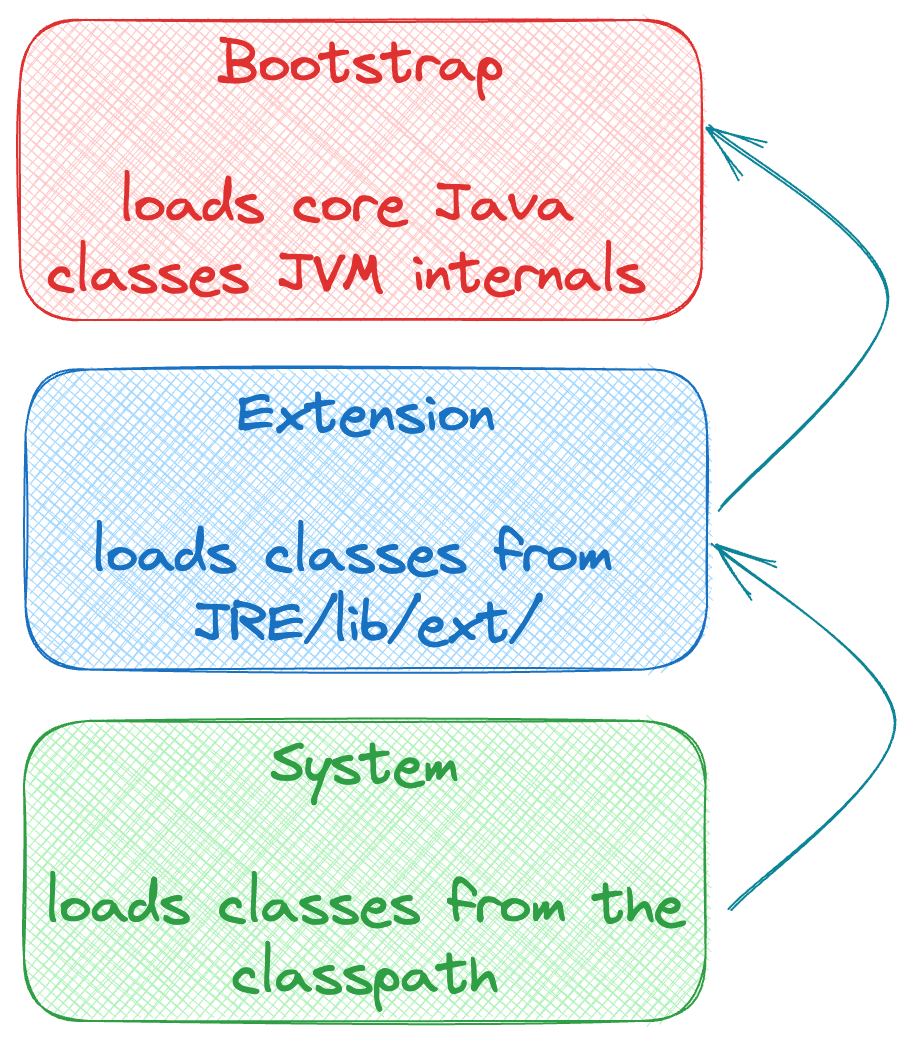
ClassLoader class
The ClassLoader
class is an abstract class that provides the basic functionality of a class loader. It is the base class for all the class loaders in Java. It is part of the java.lang
package and is loaded by the Bootstrap
class loader. Remember that the Bootstrap class loader is not a Java class, but a built-in class loader written in native code.
Custom Class Loader
Since the ClassLoader
class is abstract we can't instantiate it directly. We need to extend it and override the findClass()
method. So it is possible to implement our own custom class loader. This is useful when we want to load classes from a different source than the classpath. For example, we can load classes from a database.
Conclusion
The Java Class Loader is a great feature of the JVM. Most of the time we don't need to worry about it, but it is good to know how it works under the hood.